本文介绍了如何在MQL4中创建和管理按钮对象,以及如何利用各种函数来设置按钮的属性和行为。通过详细讨论和实例演示,您将了解如何使用MQL4语言在MetaTrader 4平台上实现图表中的交互式按钮。
按钮对象在MQL4中扮演着重要角色,可用于增强交易系统的用户界面,使交易员能够快速执行操作而无需直接干预代码。
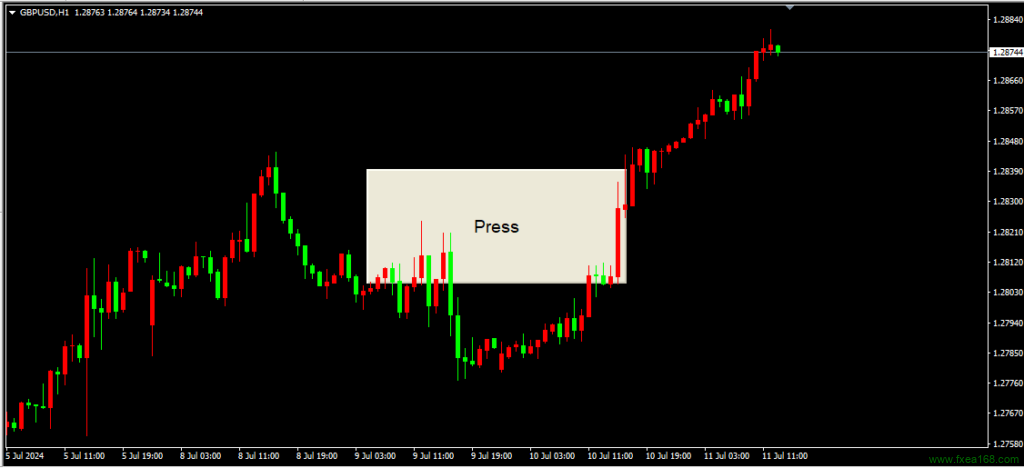
创建按钮对象
按钮对象的创建涉及到多个属性的设置,如位置、大小、文本、字体样式和颜色等。以下是创建按钮的基本步骤:
bool ButtonCreate(const long chart_ID=0,
const string name="Button",
const int sub_window=0,
const int x=0,
const int y=0,
const int width=50,
const int height=18,
const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER,
const string text="Button",
const string font="Arial",
const int font_size=10,
const color clr=clrBlack,
const color back_clr=C'236,233,216',
const color border_clr=clrNONE,
const bool state=false,
const bool back=false,
const bool selection=false,
const bool hidden=true,
const long z_order=0)
{
// 重置错误值
ResetLastError();
// 创建按钮
if(!ObjectCreate(chart_ID, name, OBJ_BUTTON, sub_window, 0, 0))
{
Print(__FUNCTION__, ": 创建按钮失败!错误代码 = ", GetLastError());
return false;
}
// 设置按钮属性
ObjectSetInteger(chart_ID, name, OBJPROP_XDISTANCE, x);
ObjectSetInteger(chart_ID, name, OBJPROP_YDISTANCE, y);
ObjectSetInteger(chart_ID, name, OBJPROP_XSIZE, width);
ObjectSetInteger(chart_ID, name, OBJPROP_YSIZE, height);
ObjectSetInteger(chart_ID, name, OBJPROP_CORNER, corner);
ObjectSetString(chart_ID, name, OBJPROP_TEXT, text);
ObjectSetString(chart_ID, name, OBJPROP_FONT, font);
ObjectSetInteger(chart_ID, name, OBJPROP_FONTSIZE, font_size);
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
ObjectSetInteger(chart_ID, name, OBJPROP_BGCOLOR, back_clr);
ObjectSetInteger(chart_ID, name, OBJPROP_BORDER_COLOR, border_clr);
ObjectSetInteger(chart_ID, name, OBJPROP_STATE, state);
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
// 成功执行
return true;
}
移动和修改按钮
除了创建按钮外,还可以通过函数来移动按钮、更改按钮的大小、修改按钮的文本内容以及调整按钮锚定的角落位置。以下是几个示例函数:
- 移动按钮:
ButtonMove
函数用于改变按钮的位置。 - 改变按钮大小:
ButtonChangeSize
函数用于调整按钮的宽度和高度。 - 改变按钮文本:
ButtonTextChange
函数允许修改按钮显示的文本内容。 - 改变按钮锚点角落:
ButtonChangeCorner
函数用于调整按钮在图表中的锚定角落位置。
这些函数不仅简化了按钮对象的管理,还使得在交易系统中动态调整和控制按钮行为变得更加灵活和便捷。
示例应用
下面是一个简单的示例,展示了如何创建、移动和最终删除按钮对象:
void OnStart()
{
// 获取图表尺寸
long x_distance, y_distance;
if(!ChartGetInteger(0, CHART_WIDTH_IN_PIXELS, 0, x_distance))
{
Print("获取图表宽度失败!错误代码 = ", GetLastError());
return;
}
if(!ChartGetInteger(0, CHART_HEIGHT_IN_PIXELS, 0, y_distance))
{
Print("获取图表高度失败!错误代码 = ", GetLastError());
return;
}
// 设置按钮初始位置和尺寸
int x = (int)x_distance / 32;
int y = (int)y_distance / 32;
int x_size = (int)x_distance * 15 / 16;
int y_size = (int)y_distance * 15 / 16;
// 创建按钮
if(!ButtonCreate(0, "MyButton", 0, x, y, x_size, y_size, CORNER_RIGHT_LOWER, "点击", "Arial", 14,
clrBlack, C'236,233,216', clrNONE, false, false, true, true, 0))
{
return;
}
ChartRedraw();
Sleep(3000); // 等待3秒钟
// 移动按钮并改变大小
x += (int)x_distance / 4;
y += (int)y_distance / 4;
x_size /= 2;
y_size /= 2;
if(!ButtonMove(0, "MyButton", x, y))
return;
if(!ButtonChangeSize(0, "MyButton", x_size, y_size))
return;
ChartRedraw();
Sleep(2000); // 等待2秒钟
// 删除按钮
ButtonDelete(0, "MyButton");
ChartRedraw();
}
通过本文,您学习了如何利用MQL4语言创建、管理和控制按钮对象。这些技术不仅能增强交易系统的可操作性和用户体验,还能提高交易决策的效率和准确性。如果您想进一步深入了解如何利用按钮对象增强您的交易策略,建议继续探索MQL4的各种功能和API文档。