本文将详细介绍如何在MQL4中创建和管理标签对象(Label Object),并且深入探讨如何使用不同的属性和函数来定制和操作这些对象。
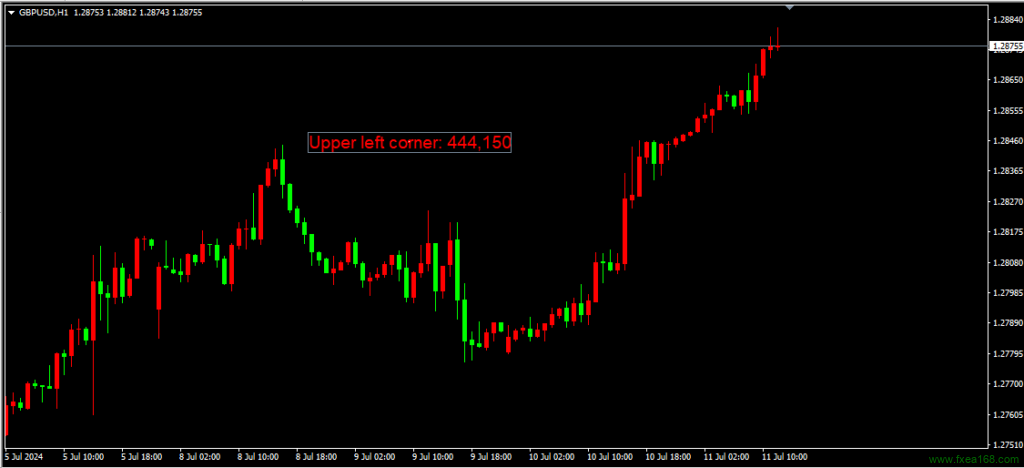
标签对象概述
标签对象(Label Object)是MetaTrader 4平台中的一种图形对象,用于在交易图表上显示文本信息。通过标签对象,您可以精确控制文本的位置、字体、颜色以及与图表窗口边角的相对关系。标签对象通常用于显示价格标签、技术指标值或自定义注释,以辅助交易决策或分析市场走势。
枚举和属性
在MQL4中,标签对象的创建和定位依赖于以下关键属性:
- OBJPROP_CORNER:指定标签对象相对于图表的哪个角落定位。
- OBJPROP_ANCHOR:定义标签对象相对于自身的锚点位置。
- OBJPROP_TEXT:设置标签对象显示的文本内容。
- OBJPROP_FONT 和 OBJPROP_FONTSIZE:控制文本的字体和大小。
- OBJPROP_COLOR:指定文本的颜色。
- OBJPROP_XDISTANCE 和 OBJPROP_YDISTANCE:设置标签对象的X和Y坐标位置。
示例代码解析
以下是一个示例脚本,演示了如何创建、移动和更改标签对象的属性:
#property strict
#property description "Script creates \"Label\" graphical object."
input string InpName="Label"; // 标签名称
input int InpX=150; // X轴距离
input int InpY=150; // Y轴距离
input string InpFont="Arial"; // 字体
input int InpFontSize=14; // 字体大小
input color InpColor=clrRed; // 颜色
input double InpAngle=0.0; // 倾斜角度
input ENUM_ANCHOR_POINT InpAnchor=ANCHOR_CENTER; // 锚点类型
input bool InpBack=false; // 背景对象
input bool InpSelection=true; // 高亮显示以移动
input bool InpHidden=true; // 在对象列表中隐藏
input long InpZOrder=0; // 鼠标点击优先级
// 创建标签对象
bool LabelCreate(const long chart_ID=0,
const string name="Label",
const int sub_window=0,
const int x=0,
const int y=0,
const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER,
const string text="Label",
const string font="Arial",
const int font_size=10,
const color clr=clrRed,
const double angle=0.0,
const ENUM_ANCHOR_POINT anchor=ANCHOR_LEFT_UPPER,
const bool back=false,
const bool selection=false,
const bool hidden=true,
const long z_order=0)
{
// 重置错误状态
ResetLastError();
// 创建文本标签
if(!ObjectCreate(chart_ID, name, OBJ_LABEL, sub_window, 0, 0))
{
Print(__FUNCTION__, ": 创建文本标签失败!错误代码 = ", GetLastError());
return false;
}
// 设置标签坐标
ObjectSetInteger(chart_ID, name, OBJPROP_XDISTANCE, x);
ObjectSetInteger(chart_ID, name, OBJPROP_YDISTANCE, y);
// 设置锚点类型
ObjectSetInteger(chart_ID, name, OBJPROP_ANCHOR, anchor);
// 设置文本内容
ObjectSetString(chart_ID, name, OBJPROP_TEXT, text);
// 设置字体
ObjectSetString(chart_ID, name, OBJPROP_FONT, font);
// 设置字体大小
ObjectSetInteger(chart_ID, name, OBJPROP_FONTSIZE, font_size);
// 设置文本颜色
ObjectSetInteger(chart_ID, name, OBJPROP_COLOR, clr);
// 设置倾斜角度
ObjectSetDouble(chart_ID, name, OBJPROP_ANGLE, angle);
// 设置背景显示
ObjectSetInteger(chart_ID, name, OBJPROP_BACK, back);
// 设置是否可选择移动
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTABLE, selection);
ObjectSetInteger(chart_ID, name, OBJPROP_SELECTED, selection);
// 设置是否隐藏在对象列表中
ObjectSetInteger(chart_ID, name, OBJPROP_HIDDEN, hidden);
// 设置鼠标点击优先级
ObjectSetInteger(chart_ID, name, OBJPROP_ZORDER, z_order);
// 操作成功
return true;
}
// 移动文本标签
bool LabelMove(const long chart_ID=0,
const string name="Label",
const int x=0,
const int y=0)
{
// 重置错误状态
ResetLastError();
// 移动文本标签
if(!ObjectSetInteger(chart_ID, name, OBJPROP_XDISTANCE, x))
{
Print(__FUNCTION__, ": 移动X坐标失败!错误代码 = ", GetLastError());
return false;
}
if(!ObjectSetInteger(chart_ID, name, OBJPROP_YDISTANCE, y))
{
Print(__FUNCTION__, ": 移动Y坐标失败!错误代码 = ", GetLastError());
return false;
}
// 操作成功
return true;
}
// 修改标签对象的角落位置
bool LabelChangeCorner(const long chart_ID=0,
const string name="Label",
const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER)
{
// 重置错误状态
ResetLastError();
// 修改锚点角落位置
if(!ObjectSetInteger(chart_ID, name, OBJPROP_CORNER, corner))
{
Print(__FUNCTION__, ": 修改锚点角落失败!错误代码 = ", GetLastError());
return false;
}
// 操作成功
return true;
}
// 修改标签对象的文本内容
bool LabelTextChange(const long chart_ID=0,
const string name="Label",
const string text="Text")
{
// 重置错误状态
ResetLastError();
// 修改对象文本
if(!ObjectSetString(chart_ID, name, OBJPROP_TEXT, text))
{
Print(__FUNCTION__, ": 修改文本失败!错误代码 = ", GetLastError());
return false;
}
// 操作成功
return true;
}
// 删除文本标签
bool LabelDelete(const long chart_ID=0,
const string name="Label")
{
// 重置错误状态
ResetLastError();
// 删除标签
if(!ObjectDelete(chart_ID, name))
{
Print(__FUNCTION__, ": 删除文本标签失败!错误代码 = ", GetLastError());
return false;
}
// 操作成功
return true;
}
// 脚本程序开始函数
void OnStart()
{
// 存储标签的坐标
int x = InpX;
int y = InpY;
// 获取图表窗口尺寸
long x_distance, y_distance;
if(!ChartGetInteger(0, CHART_WIDTH_IN_PIXELS, 0, x_distance))
{
Print("获取图表宽度失败!错误代码 = ", GetLastError());
return;
}
if(!ChartGetInteger(0, CHART_HEIGHT_IN_PIXELS, 0, y_distance))
{
Print("获取图表高度失败!错误代码 = ", GetLastError());
return;
}
// 检查输入参数的正确性
if(InpX < 0 || InpX > x_distance - 1 || InpY < 0 || InpY > y_distance - 1)
{
Print("错误!输入参数值不正确!");
return;
}
// 准备标签的初始文本
string text = StringConcatenate("左上角: ", x, ",", y);
// 在图表上创建文本标签
if(!LabelCreate(0, InpName, 0, InpX, InpY, CORNER_LEFT_UPPER, text, InpFont, InpFontSize,
InpColor, Inp
Angle, InpAnchor, InpBack, InpSelection, InpHidden, InpZOrder))
{
return;
}
// 重绘图表并等待半秒钟
ChartRedraw();
Sleep(500);
// 向下移动标签并同时修改其文本
int h_steps = (int)(x_distance / 2 - InpX);
int v_steps = (int)(y_distance / 2 - InpY);
for(int i = 0; i < v_steps; i++)
{
// 改变坐标
y += 2;
// 移动标签并修改文本
MoveAndTextChange(x, y, "左上角: ");
}
Sleep(500);
// 向右移动标签
for(int i = 0; i < h_steps; i++)
{
// 改变坐标
x += 2;
// 移动标签并修改文本
MoveAndTextChange(x, y, "左上角: ");
}
Sleep(500);
// 向上移动标签
for(int i = 0; i < v_steps; i++)
{
// 改变坐标
y -= 2;
// 移动标签并修改文本
MoveAndTextChange(x, y, "左上角: ");
}
Sleep(500);
// 向左移动标签
for(int i = 0; i < h_steps; i++)
{
// 改变坐标
x -= 2;
// 移动标签并修改文本
MoveAndTextChange(x, y, "左上角: ");
}
Sleep(500);
// 现在,通过更改锚点角落移动标签
// 移动到左下角
if(!LabelChangeCorner(0, InpName, CORNER_LEFT_LOWER))
return;
// 更改标签文本
text = StringConcatenate("左下角: ", x, ",", y);
if(!LabelTextChange(0, InpName, text))
return;
ChartRedraw();
Sleep(2000);
// 移动到右下角
if(!LabelChangeCorner(0, InpName, CORNER_RIGHT_LOWER))
return;
// 更改标签文本
text = StringConcatenate("右下角: ", x, ",", y);
if(!LabelTextChange(0, InpName, text))
return;
ChartRedraw();
Sleep(2000);
// 移动到右上角
if(!LabelChangeCorner(0, InpName, CORNER_RIGHT_UPPER))
return;
// 更改标签文本
text = StringConcatenate("右上角: ", x, ",", y);
if(!LabelTextChange(0, InpName, text))
return;
ChartRedraw();
Sleep(2000);
// 移动到左上角
if(!LabelChangeCorner(0, InpName, CORNER_LEFT_UPPER))
return;
// 更改标签文本
text = StringConcatenate("左上角: ", x, ",", y);
if(!LabelTextChange(0, InpName, text))
return;
ChartRedraw();
Sleep(2000);
// 删除标签
LabelDelete(0, InpName);
ChartRedraw();
Sleep(500);
}
// 移动对象并修改文本的功能
bool MoveAndTextChange(const int x, const int y, string text)
{
// 移动标签
if(!LabelMove(0, InpName, x, y))
return false;
// 更改标签文本
text = StringConcatenate(text, x, ",", y);
if(!LabelTextChange(0, InpName, text))
return false;
// 重绘图表
ChartRedraw();
// 等待0.01秒
Sleep(10);
// 函数结束
return true;
}
结论
通过本文,您学习了如何在MQL4中使用标签对象创建、定位和管理文本标签。这些技术可以帮助您更好地管理交易图表上的信息展示,从而提升交易决策的效率和准确性。在实际应用中,您可以根据具体需求调整标签对象的属性,以实现定制化的交易辅助功能。